Master Destructuring Assignment in JavaScript: Simplify Your Code with Unpacking
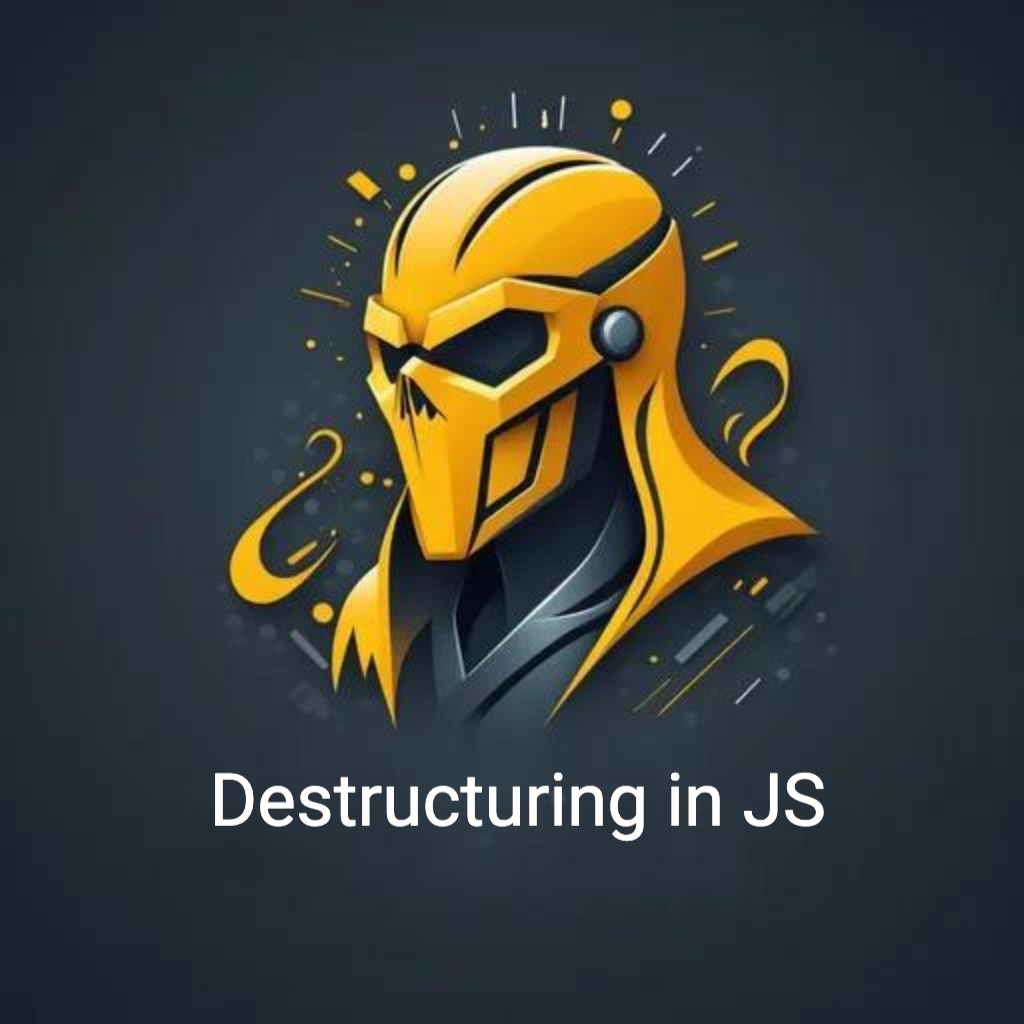
Let’s imagine that we have an object which we want to access. This object will look like this:
let req = {
params: {
id: 'Request params ID',
params: {
id: 'Internal ID'
}
},
body: 'Request body'
};
To access elements of this object we can:
req.params;
req.body;
To display them in console:
console.log(req.params);
console.log(req.body);
In case we want to assign those properties into variables or consts:
const params = req.params;
const body = req.body;
So in case this object had more properties like:
let req = {
params: {
id: 'Request params ID',
params: {
id: 'Internal ID'
}
},
body: 'Request body',
param2: 'value of param2',
param3: 'value of param3',
param4: 'value of param4',
};
and we would like to assign them to variables/consts we would need to have:
const params = req.params;
const body = req.body;
const param2 = req.param2;
const param3 = req.param3;
const param4 = req.params4;
Using Destructuring assignment to assign values to variables/consts
In this case we can use Destructuring assignment:
const { params, body, param2, param3, param4 } = req;
Now object req is estructuring and const params will be equal req.params
To check how it works let’s run this code:
let req = {
params: {
id: 'Request params ID',
params: {
id: 'Internal ID'
}
},
body: 'Request body',
param2: 'value of param2',
param3: 'value of param3',
param4: 'value of param4',
};
const { params, body, param2, param3, param4 } = req;
console.log('params: ', params); // { id: 'Request params ID', params: { id: 'Internal ID' } }
console.log('body: ', body); // 'Request body'
console.log('param2: ', param2); // 'value of param2'
console.log('param3: ', param3); // 'value of param3'
console.log('param4: ', param4); // 'value of param4'
Destructuring assignment nested elements in object
Lets imagine that we need to access nested element. In this case we will try to access id value which is nested in params. Normally we would need to make it using req.body.id. Lets try to make it with Destructuring assignment
let req = {
params: {
id: 'Request params ID',
params: {
id: 'Internal ID'
}
},
body: 'Request body',
};
const { params: {id} } = req;
console.log('id: ', id); // 'Request params ID'
This will return ‘Request params ID’
Destructuring assignment and undefined elements in object
Lets imagine that we are now using Destructuring assignment but our object is not “complete”.
let req = {
params: {
id: 'Request params ID',
params: {
id: 'Internal ID'
}
},
body: 'Request body',
// param2: 'value of param2', // param2 doesn't exist
param3: 'value of param3',
param4: 'value of param4',
};
const { params, body, param2, param3, param4 } = req;
console.log('param2: ', param2); // undefined
If element doesn’t exist it will return undefined value;
Destructuring assignment and undefined nested elements in object
Lets imagine that we are now using Destructuring assignment for object:
let req = {
// params: {
// id: 'Request params ID',
// params: {
// id: 'Internal ID'
// }
// },
body: 'Request body',
// param2: 'value of param2', // param2 doesn't exist
param3: 'value of param3',
param4: 'value of param4',
};
const { params: {id}, body, param2, param3, param4 } = req;
console.log('id: ', id); // undefined
If element doesn’t exist it will return error:
Uncaught TypeError: Cannot destructure property `id` of ‘undefined’ or ‘null’.;