Conditional operator (ternary operator) in JavaScript (or TypeScript)
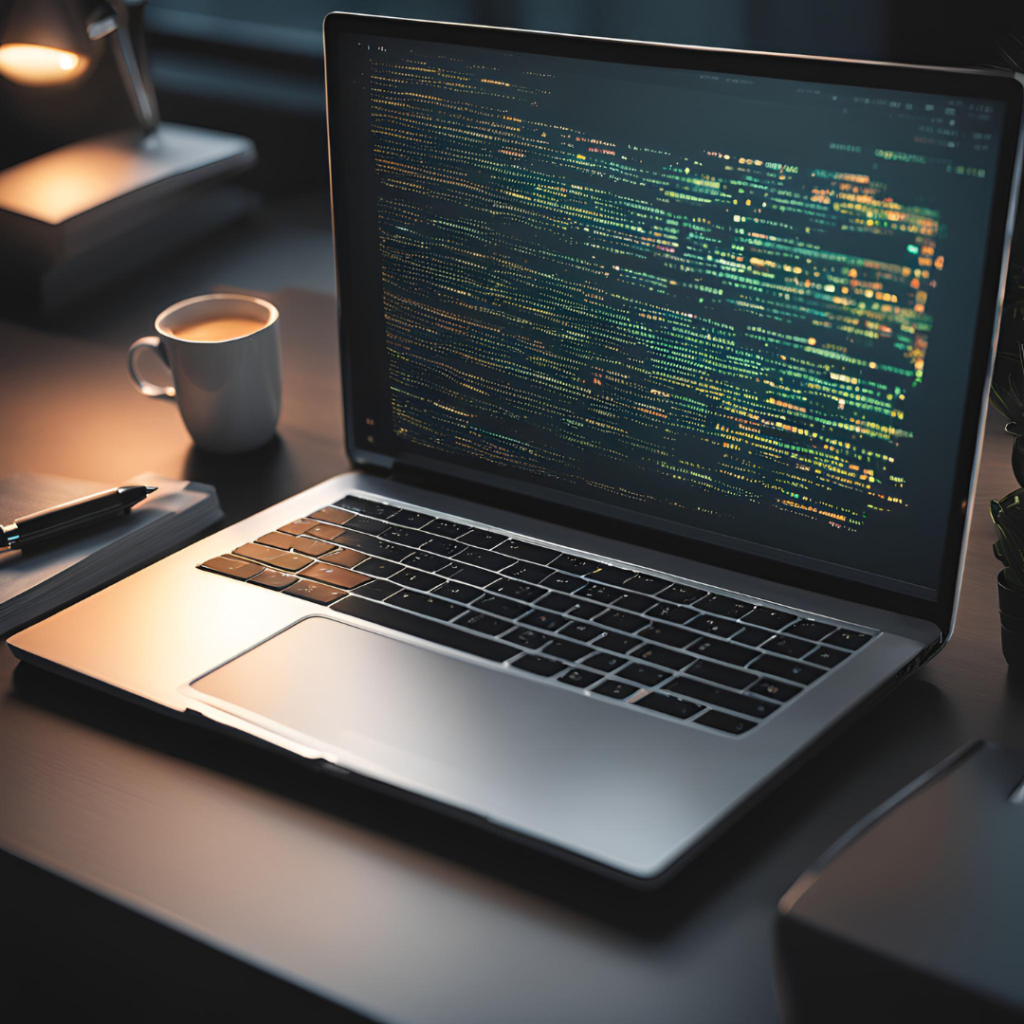
Read Time:43 Second
Let’s imagine that we could optimize / minify if statement. What could we do?
In case we have if…else statement like:
if (condition) {
// do sth if condition is true
}
else {
// do sth else if condition isn't true
}
we can change it to ternary expression
condition ? /* do sth if condition is true */ : /* do sth if condition isn't true */;
Let’s check how it works with functions:
const actionOne = () => console.log('actionOne');
const actionTwo = () => console.log('actionTwo');
true ? actionOne() : actionTwo(); // actionOne
false ? actionOne() : actionTwo(); // actionTwo
And how to use it with more complex functions:
const multiply = (a) => a*a;
const add = (a) => a+a;
const variable = 4;
let newVar;
newVar = true ? multiply(variable) : add(variable);
console.log(newVar) // 16
newVar = false ? multiply(variable) : add(variable);
console.log(newVar) // 8
As you can see ternary can be useful when you want to make inline if operations.